二叉树的遍历 | Go基础
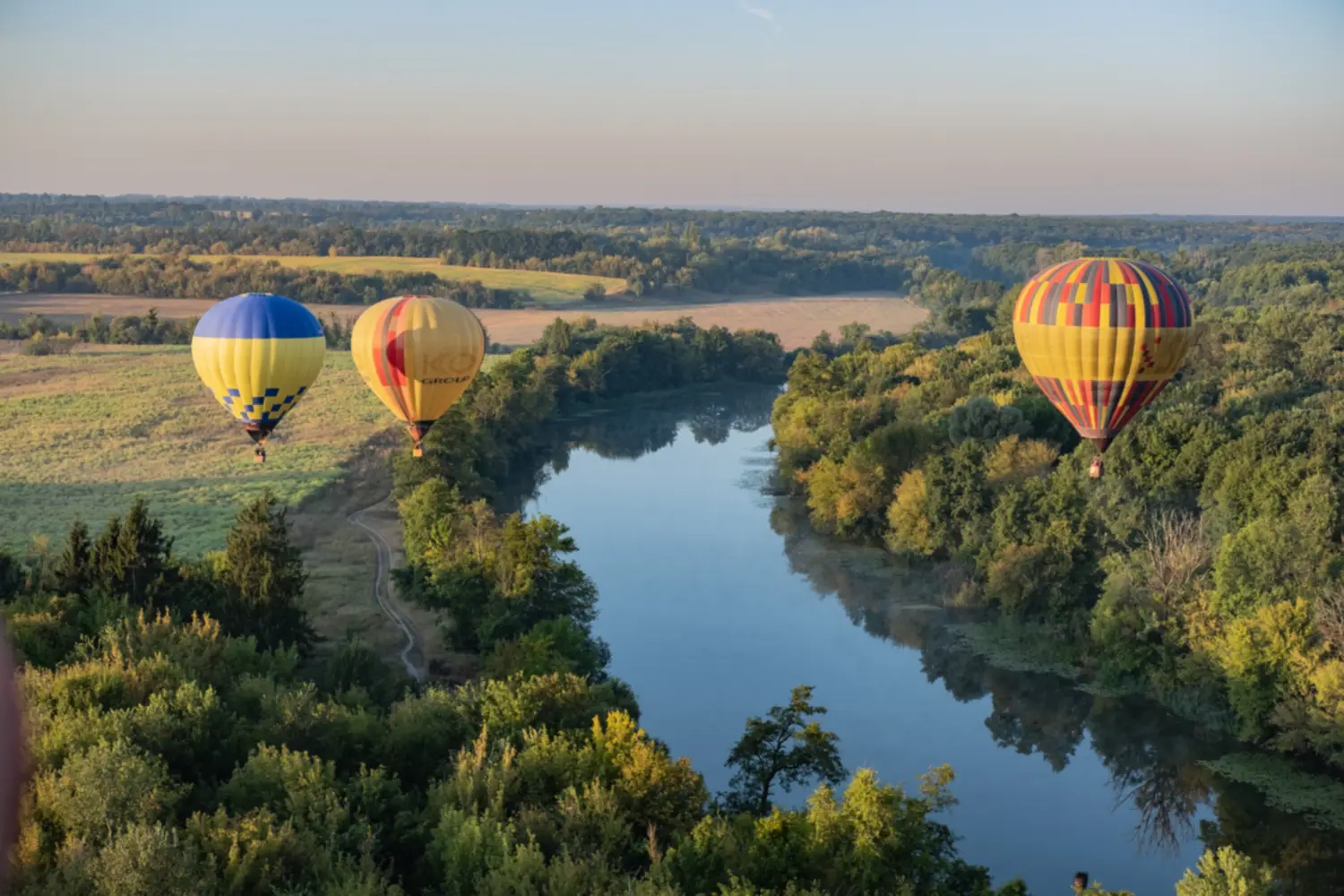
前言
用Go语言复习下二叉树的遍历:
- 前序
- 中序
- 后序
- 层序
准备
一个简单的二叉树:
1 |
推理出,理论输出:
前序输出: 1 2 4 5 3 6 8 9 7 |
代码:
// Tree 二叉树的基础结构体 |
// 初始化这个简单的二叉树 |
遍历
前序
// 前序 |
输出:
[Running] go run "../easy-tips/go/src/algorithm/tree.go" |
中序
// 中序 |
输出:
[Running] go run "../easy-tips/go/src/algorithm/tree.go" |
后序
// 后序 |
输出:
[Running] go run "../easy-tips/go/src/algorithm/tree.go" |
层序
使用队列达到有序的目的。
// Queue 队列 |
输出:
[Running] go run "../easy-tips/go/src/algorithm/tree.go" |
结语
本文便于,后续回忆复习使用。
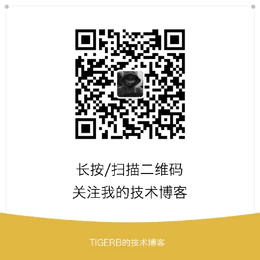